2. Dynamic Building Interfaces (witkets.tkbuilder.TkBuilder
)¶
2.1. Purpose¶
This class is responsible for constructing Tk user interfaces based on XML descriptions. It is somewhat similar to GtkBuilder, but without Glade (yet).
2.2. Basic Usage¶
Consider the following XML string:
demo2.xml
<?xml version="1.0"?>
<root>
<label wid="lbl1" text="Cap. Kirk" background="red" />
<frame wid="frm1">
<label wid="lbl2" text="Bones" />
<label wid="lbl3" text="Spock" />
<geometry>
<grid for="lbl2" row="0" column="0" sticky="w" />
<grid for="lbl3" row="0" column="2" sticky="e"/>
</geometry>
</frame>
<geometry>
<pack for="lbl1" fill="y" expand="1" />
<pack for="frm1" />
</geometry>
</root>
It describes children of a given Tk container (root element). For instance, when
building the interface, TkBuilder
will insert a label, which can be
accessed later by the identifier lbl1. This label will have the text property
set to Cap. Kirk and its background property set to red. In fact, for a
given object obj
, most of the XML attributes (the wid being an
exception) are treated just like this:
obj[attrName] = attrValue
The special (and required) wid attribute is used for retrieving objects from the builder once the GUI has been constructed. The special geometry tag is used to define the GUI layout based on the Tk geometry managers: pack, grid and place. The geometry tag must appear after the definition of all its referenced widgets, just like the example above. In other words, it probably should be the last child of a given container.
The Python snippet below should just build the interface described by demo1.xml into the main Tk frame:
demo2.py
#!/usr/bin/env python3
import tkinter as tk
import witkets as wtk
root = tk.Tk()
builder = wtk.TkBuilder(root)
builder.build_from_file('demo2.xml')
root.mainloop()
The builded GUI is shown below:
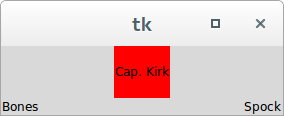
2.3. Accessing the Widgets¶
After building the interface, you can access each widget with the nodes attribute:
label = builder['nodes']['lbl1']
print(label['text']) # Cap. Kirk
2.5. Theme Specification¶
A style tag can appear as a child of the root tag, requiring the theme’s INI specification to be either inside the tag or referenced to a file by the fromfile attribute. In this second case, the path is relative to working directory of Python. Also, if the attribute defaultfonts is defined and not ‘0’, all widget fonts will be set to ‘Helvetica 11’. Finally, if the attribute applydefault is given with some value other than ‘0’ the default theme is applied.
For further details, please refer to the section Themes and Styling.
2.5.1. Example¶
<root>
<style defaultfonts="1">
[SpockLabel.TLabel]
foreground=#088
background=#000
</style>
<!--as the first XML example...-->
<label wid="lbl3" text="Spock" style="SpockLabel.TLabel" />
<!--as the first XML example...-->
</root>
<root>
<style fromfile="styles.ini" />
<!--your layout here-->
</root>
2.6. Visual Designers¶
A visual designer named tksimplegui will eventually be released with limited supported for witkets XML.
2.7. API Reference¶
- class witkets.TkBuilder(master)¶
- __init__(master)¶
Initializer
- Parameters
master – Tk root container where interface is going to be built
- add_tag(tag: str, cls, container=False)¶
Maps a tag to a class.
- Parameters
tag (str) – XML tag name
cls (Any widget class) – Class to be instantiated when tag is found
container – Whether this Tk widget is a container of other widgets
- build_from_file(filepath)¶
Build user interface from XML file.
- build_from_string(contents)¶
Build user interface from XML string
- set_custom_attribute(tag: str, attribute: str, handler)¶
Set a handler for a custom (user-defined) attribute.
- Parameters
tag (str) – XML tag name.
attribute (str) – XML attribute name.
handler – Handler function with signature f(widget, key, strval).
- set_custom_child_tag(tag: str, child_tag: str, handler)¶
Set a handler for a custom (user-defined) child tag.
- Parameters
tag (str) – XML tag name.
child_tag – The XML tag to be registered for this widget.
handler – Handler function with signature f(widget, child_node).